An intro to HTML
What is HTML?
HTML stands for HyperText Markup Language. HTML is used on the web in order to "markup", or format, pieces of text. Instead of being plain-text, this allows the text to be in italics, bold, and have all other cool stuff. In this lesson I will talk about the basics as well some useful sources of information about HTML
To create and run HTML files, open up a text editor (such as notepad) and enter all the HTML. When you are done, click save as and put: exampleFileName.html
When you run the HTML file, it should open up in your browser.
HTML: All about those tags
In HTML text is formatted by enclosing the text in tags. For example, <h1> is a type of tag. Most tags have a beginning and end tag. The beginning tag is <x> where x is the name of the tag and the closing tag is formatted like: </x>
So, for example, let's say we had a normal piece of text like
Hello, World!
Not so interesting, is it? But... if we surround it with the tag <i> it will be in italics.
<i>Hello, World!</i> when run in your browser looks like:
<i>Hello, World!</i> when run in your browser looks like:
<b>Hello, world</b> when run in your browser looks like:
What if we want to make the text both bold and italicized? We can combine tags to do that!
For example:
<b><i>Hello, World!</i></b>
Will give you:
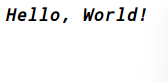
More Info
HTML dog
HTML Tags List
Code Academy HTML tutorial